C The Mother of Programming Language
2025-01-01
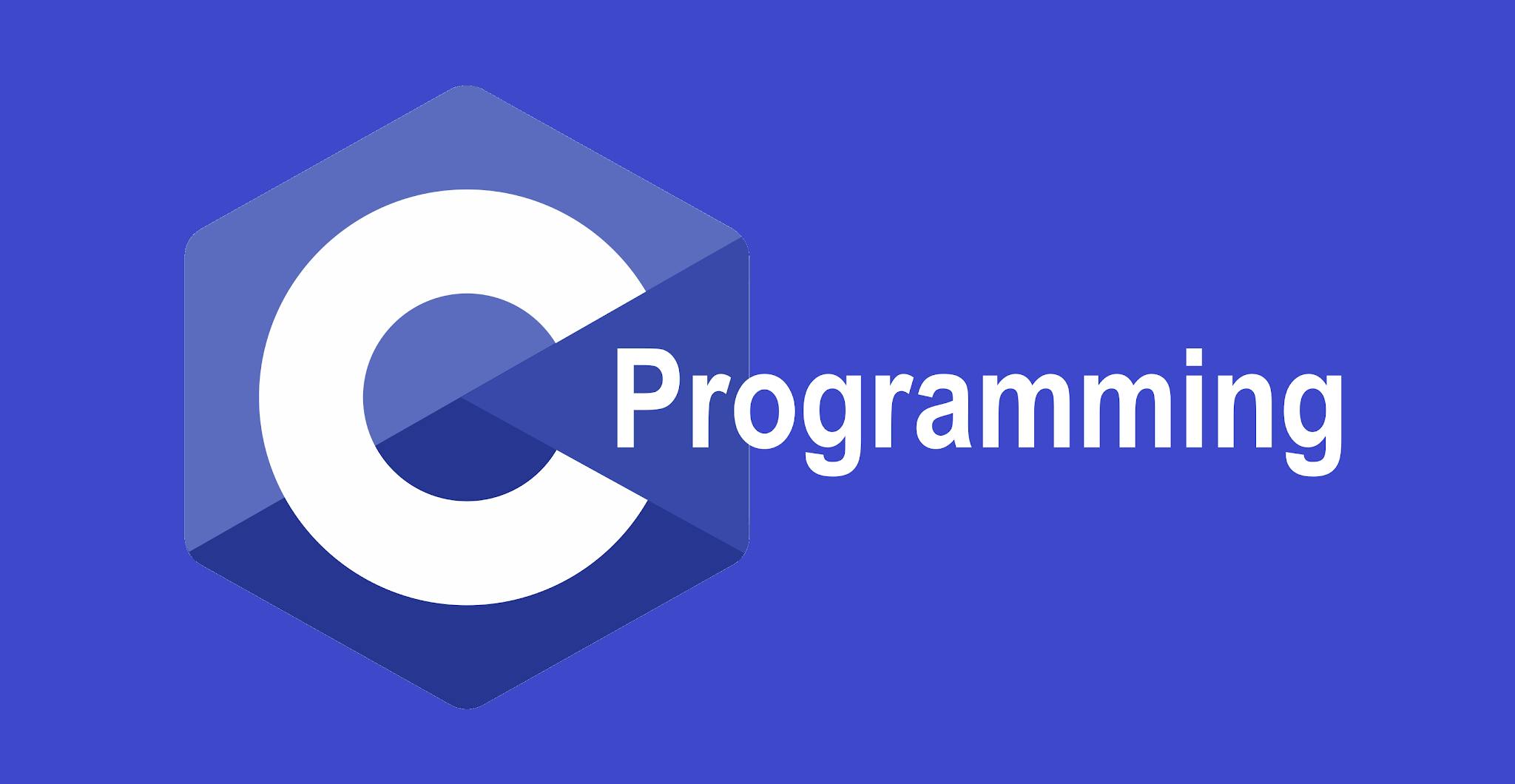
C Programming Language: A Comprehensive Guide
C is a powerful, versatile, and efficient programming language that has stood the test of time. Developed by Dennis Ritchie in the early 1970s, C is widely used for system programming, embedded systems, and application development. In this blog, we’ll delve into the pros and cons of C, its learning benefits, and explore advanced topics like pointers, dynamic memory allocation, and file manipulation.
Pros and Cons of C Programming
Pros:
- Portability: Programs written in C are highly portable, meaning they can run on various platforms with minimal changes.
- Performance: C is a compiled language that offers high performance and low-level access to memory.
- Simplicity: Despite its power, C has a relatively simple syntax, making it easy to understand and use.
- Versatility: From system-level programming to game development, C can be used for a wide variety of applications.
- Extensive Library Support: C comes with a rich set of libraries for performing various tasks.
Cons:
- Manual Memory Management: While it provides control, managing memory manually can lead to errors like memory leaks.
- Lack of Object-Oriented Features: Unlike modern languages like C++ or Java, C lacks built-in support for object-oriented programming.
- No Garbage Collection: Developers need to explicitly allocate and deallocate memory.
- Steep Learning Curve for Advanced Topics: Concepts like pointers and bitwise operations can be challenging for beginners.
Learning Benefits of C Programming
- Foundation for Other Languages: Learning C provides a strong foundation for understanding other programming languages like C++, Java, and Python.
- System-Level Knowledge: C enables you to work closely with hardware, which is invaluable for system programming and embedded development.
- Problem-Solving Skills: Writing efficient C programs helps sharpen your problem-solving skills.
- Career Opportunities: C is still widely used in industries like embedded systems, robotics, and gaming.
Pointers and Dynamic Memory Allocation
Pointers:
Pointers are one of the most powerful features of C. A pointer is a variable that stores the memory address of another variable. Pointers allow direct memory access, which is essential for tasks like dynamic memory allocation, data structures (linked lists, trees), and file handling.
Example:
#include <stdio.h> int main() { int x = 10; int *ptr = &x; // Pointer to x printf("Value of x: %d\n", x); printf("Address of x: %p\n", ptr); printf("Value at address: %d\n", *ptr); return 0; }
Dynamic Memory Allocation:
Dynamic memory allocation allows programs to request memory at runtime using functions like malloc, calloc, realloc, and free.
Example:
#include <stdio.h> #include <stdlib.h> int main() { int *arr; int n = 5; arr = (int *)malloc(n * sizeof(int)); // Allocating memory for 5 integers if (arr == NULL) { printf("Memory allocation failed\n"); return 1; } for (int i = 0; i < n; i++) { arr[i] = i + 1; } for (int i = 0; i < n; i++) { printf("%d ", arr[i]); } free(arr); // Freeing allocated memory return 0; }
File Handling in C
C provides robust support for file handling, enabling developers to create, read, write, and manipulate files. Common functions include fopen, fclose, fread, fwrite, and fprintf.
Example: Reading a Text File
#include <stdio.h> int main() { FILE *file; char buffer[255]; file = fopen("example.txt", "r"); if (file == NULL) { printf("Error opening file\n"); return 1; } while (fgets(buffer, sizeof(buffer), file)) { printf("%s", buffer); } fclose(file); return 0; }
Bitwise Operations and File Manipulation
Manipulating Bits:
C provides operators for bitwise manipulation, such as &, |, ^, ~, <<, and >>. These are useful for low-level tasks like setting or clearing specific bits in a variable.
Example: Checking if a Number is Odd or Even
#include <stdio.h> int main() { int num = 5; if (num & 1) { printf("%d is odd\n", num); } else { printf("%d is even\n", num); } return 0; }
Manipulating Binary Files:
C allows reading and writing binary files, which is essential for tasks like image processing or encryption.
Example: Writing and Reading a Binary File
#include <stdio.h> int main() { FILE *file; int num = 12345; file = fopen("data.bin", "wb"); fwrite(&num, sizeof(num), 1, file); fclose(file); file = fopen("data.bin", "rb"); fread(&num, sizeof(num), 1, file); printf("Read from file: %d\n", num); fclose(file); return 0; }
Conclusion
C remains one of the most important programming languages for developers to learn. Its simplicity, power, and performance make it ideal for system programming and application development. While mastering C involves understanding complex topics like pointers, dynamic memory allocation, and file manipulation, the benefits far outweigh the challenges. Whether you're a beginner or an experienced developer, C will equip you with skills that are valuable in any programming field.