React Core
2024-04-15
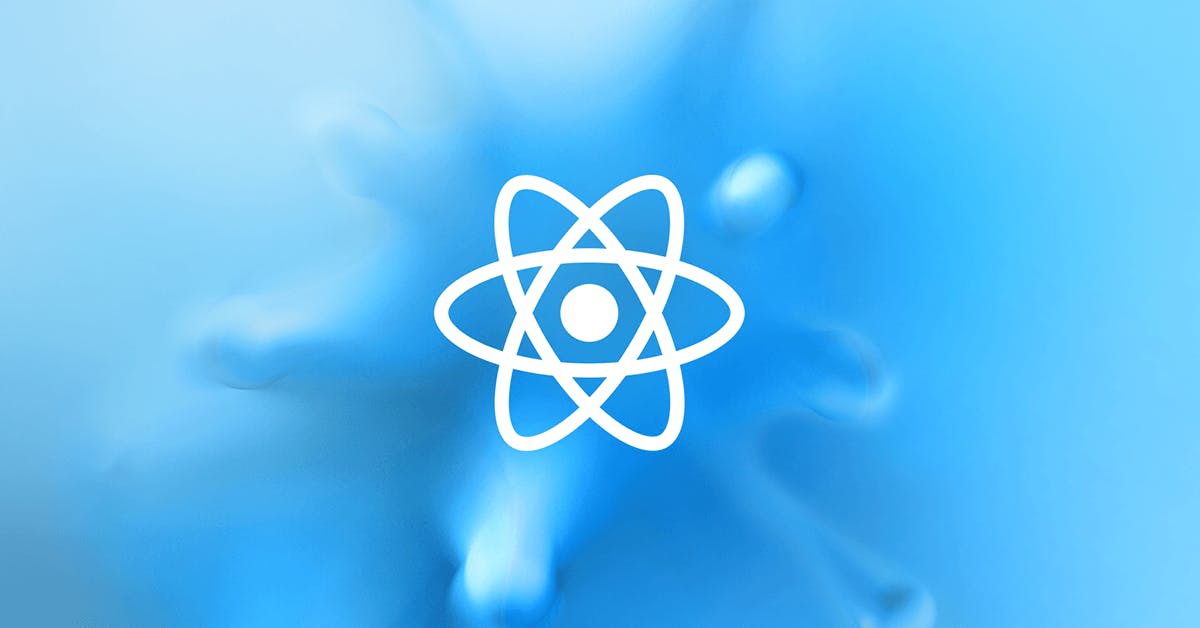
Understanding Core Concepts of React
React, a JavaScript library developed by Facebook, has revolutionized the way developers build user interfaces. Known for its efficiency, flexibility, and declarative nature, React has become a cornerstone in modern web development. In this article, we will delve into the core concepts of React that every developer should understand to build robust and scalable applications.
1. Components
Definition: Components are the building blocks of a React application. They encapsulate pieces of the UI, logic, and state.
Types of Components:
- Functional Components: These are simple JavaScript functions that accept props and return React elements. They are also known as stateless components.
- Class Components: These are ES6 classes that extend React.Component and include a render method that returns React elements. They are also known as stateful components.
Importance: Components promote reusability, modularity, and maintainability in code. Each component manages its content, presentation, and behavior.
2. JSX
Definition: JSX (JavaScript XML) is a syntax extension for JavaScript that allows writing HTML-like code within JavaScript. JSX makes the code easier to write and understand by combining HTML and JavaScript.
Importance: JSX simplifies the process of creating and rendering elements, making the code more readable and easier to debug.
3. State and Props
State:
- Definition: State is a built-in React object used to store data about the component. It is managed within the component and can change over time.
Props:
- Definition: Props (short for properties) are read-only attributes passed from parent to child components. They allow data to flow through the application.
Importance: State and props are essential for managing data and enabling communication between components.
4. Lifecycle Methods
Definition: Lifecycle methods are special methods in class components that get called at different stages of a component’s existence, such as mounting, updating, and unmounting.
Key Lifecycle Methods:
- componentDidMount: Called after the component is rendered to the DOM. Useful for fetching data.
- componentDidUpdate: Called after the component's updates are flushed to the DOM. Useful for reacting to prop or state changes.
- componentWillUnmount: Called just before the component is removed from the DOM. Useful for cleanup tasks.
Importance: Lifecycle methods provide hooks to run code at specific times, enabling precise control over component behavior.
5. Handling Events
Definition: React uses synthetic events, which are a cross-browser wrapper around the browser’s native event. This ensures consistency in event behavior across different browsers.
Importance: Handling events in React is straightforward and similar to handling events in plain JavaScript. This helps in building interactive UIs.
6. Conditional Rendering
Definition: Conditional rendering in React works the same way conditions work in JavaScript. Use JavaScript operators like if or the ternary operator to create elements representing the current state.
Importance: Conditional rendering allows components to render different outputs based on state or props, enhancing the dynamic nature of applications.
7. Lists and Keys
Definition: Lists in React are used to render multiple items, and keys help React identify which items have changed, are added, or are removed.
Importance: Keys improve the performance of rendering lists by helping React efficiently update and manage the list elements.
8. Forms
Definition: Forms in React are used to handle user input. Controlled components, where form data is handled by the React component, are preferred.
Importance: Handling forms efficiently ensures a smooth user experience and precise control over user inputs.
Conclusion
Understanding these core concepts of React is fundamental to becoming proficient in building modern web applications. By mastering components, JSX, state and props, lifecycle methods, event handling, conditional rendering, lists and keys, and forms, developers can create interactive, efficient, and maintainable user interfaces. React’s declarative nature and component-based architecture simplify the development process, making it a powerful tool for both beginners and experienced developers.